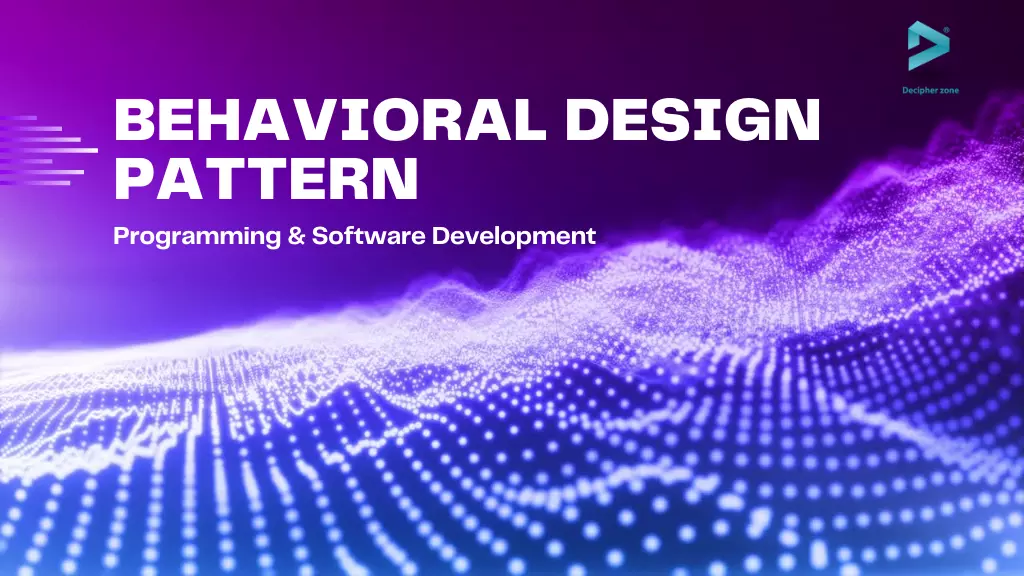
Behavioral Design Pattern in Java
Recently, we have covered Creational Patterns in our Design Patterns series. Today, we are going to cover behavioral design patterns and their types.
If you want to get an overview of design patterns before getting started with behavioral patterns, then check out our previously written blog: Design Patterns in Java.
For all those who are familiar with the concept of design patterns, let’s get started with behavioral patterns without further ado.
Behavioral Design Pattern Definition and Overview
The behavioral design pattern deals with object interactions with each other and increases flexibility in carrying out communication. It helps in categorizing how objects and classes interact to distribute responsibilities. The behavioral pattern makes it essential to keep things loosely coupled with minimum hard coding and more coherence.
Read: Custom Web Application Design Patterns
Behavioral pattern identifies the typical patterns of communication between objects and registers them. These patterns are also responsible for algorithms and responsibility assignments between objects.
Thus, the behavioral pattern uses the registered communication pattern to provide a better interaction solution between objects in a way that makes them flexible and loosely coupled.
Types of Behavioral Design Patterns
With the basic understanding of behavioral patterns that we have acquired, it is time to move ahead and learn about the types of Behavioral Design Patterns.
Read: Factory Design Patterns in Java
A behavioral pattern is a group of twelve design patterns, consisting of
-
Chain of Responsibility
-
Command Pattern
-
Interpreter Pattern
-
Iterator Pattern
-
Mediator Pattern
-
Memento Pattern
-
Observer Pattern
-
State Pattern
-
Strategy Pattern
-
Template Pattern
-
Visitor Pattern
-
Null object Pattern.
Now let’s help you understand each of them.
-
Chain of Responsibility Pattern
In this pattern, a request is sent by a sender to a chain of objects that can be handled by any object in the chain. The chain of responsibility pattern promotes minimizing coupling between the sender and receiver of the request by offering multiple objects to handle a request.
Read: When to Use Composite Design Pattern in Java
Put simply, it allows you to pass requests on a chain of handlers where each handler decides whether it will process the request or pass it to the next one. Here each handler or object in the chain contains its processing logic that upon performing some actions decides to pass the request to the next object that can complete the task.
-
Command Pattern
The command pattern encapsulates an action along with its parameters under an object as a command and passes it to the invoker object. Here the invoker object searches for the object that can handle the request and pass the command to the required object for command execution.
Read: Singleton Design Pattern
Put simply, in the command pattern, a request is converted into an independent object containing all the information. It allows developers to pass the request as method arguments, support unattainable operations, and postpone or queue the execution of the request.
-
Interpreter Pattern
It is used to define the grammar representation of a given language with an interpreter to tell the context behind the sentences.
With interpreter patterns, you get to implement a specialized language to solve a certain set of problems in the program. Using this pattern, programmers can create simpler, more human-like syntax programs.
Read: Ways To Hire Freelance Java Developers
Put simply, the interpreter pattern is used when we need to evaluate any kind of expression or grammar in any language. Some of the most popular examples of such web apps are Bing Microsoft Translator and Google Translate. In these two web apps, input is interpreted and output is shown in another language.
Another example of an interpreter pattern is the Java compiler that interprets Java code and translates it into bytecode used by Java Virtual Machine (JVM) to execute the program on the device.
Also known as Cursor, the Iterator pattern allows developers to traverse the collection’s elements without uncovering its underlying representation. Using this pattern, developers can access objects in a sequence after aggregation of the objects in a list.
It simplifies the collection interface and supports variations in the collection traversal. As the iterator pattern hides the underlying representation, accessing objects regardless of the representation in the list or tree becomes possible.
-
Mediator Pattern
A mediator pattern defines an object encapsulating the way a set of objects communicate. It lets you minimize the chaotic dependencies between objects by restricting direct interactions between objects and encourages them to collaborate using a mediator object. In short, it simplifies communication in the subsystem of an application program.
In simple terms, the mediator pattern provides a mediator class or object that handles all the interactions between classes or objects.
This pattern is useful in large-scale applications where tight coupling makes it hard to maintain, test or scale. As it promotes loose coupling, centralization control, and simplified object protocols, dealing with objects or classes becomes much easier.
-
Memento Pattern
It is used to implement undo operations in the program, i.e., with the memento pattern you get the ability to save and restore (rollback) an object to its previous state. You can use this pattern when you want to save the object’s state to restore it later.
The memento pattern helps to implement in such a way that it is not accessible outside the object to protect the data integrity. It involves two objects: The Originator and Caretaker along with an inner private class - Memento, where Originator refers to the object that you need to save and restore, and the Caretaker helps in storing and restoring the data through the Memento object.
Also known as Event Listener, the observer behavioral design pattern defines the link between objects, thus, if the state of an object changes, the states of other dependent objects are automatically updated.
It states that one should define one-to-one object dependency as it will allow dependents to be notified if there’s a change in the object’s state and update automatically. The observer pattern gives support for broadcast-type communication while describing the coupling between the observer and the objects.
In simple terms, with the observer pattern, you can define the publish/subscribe mechanism that will notify multiple objects about any changes or events that take place to the objects being observed.
-
State Pattern
When you want an object to change its behavior according to its internal state, you can use the state pattern. The state pattern offers a clean way for an object to slightly change its type/state at the time of execution. To achieve this, developers provide one or more states to each of these objects that help them change their behavior.
In this pattern, the class or object behavior varies with its state and is therefore defined by the context object. Also, with state patterns, it becomes easier to keep the object behavior state-specific and makes all state transitions clear.
-
Strategy Pattern
A strategy pattern allows you to select algorithms on the fly, i.e, it allows the algorithm to vary independently from client to client. It allows you to define the set of algorithms and arrange each of them in a different class while making the objects substitutable.
In simple terms, you can choose both algorithm and behavior of the object at the runtime, based on the user’s input.
The template method/pattern is used to define a group of similarly structured, interchangeable, multi-step algorithms. While each algorithm follows the same action series, they still provide different implementations of the steps.
Similar to the strategy pattern, the template method allows you to change the behavior of a class at the time of execution. However, there is a significant difference between both. While the strategy pattern changes the entire algorithm, with the template method, only some parts of the algorithm behavior are changed through abstract methods.
It is one of the most common methods that is used to reuse the code.
-
Visitor Pattern
Using the visitor pattern, you can separate algorithms and objects to define new operations in an existing class without changing the structure. In the visitor pattern, operations are defined in a separate class called visitor class to separate them from the complex object structure where it operates. This allows additional operations to be added to the class without changing the data class.
-
Null Object
A null object is used instead of defining a null value that acts as a default object value or conveys the absence of the object to minimize the null checks in the application program.
Takeaway
So that was all about behavioral design patterns. We hope that it helped you understand the basics of behavioral design patterns and their types along with their usage.
Remember that we have only scratched the surface of the design patterns topic and there is much more left to discover.
For every business owner out there looking for experienced and skilled professionals that can help them build their web app, you can hire developers from us at Decipher Zone Technologies. We have a team of front-end and back-end developers who are well-versed in their tools and technologies. They have also worked on multiple projects that you can check before hiring.
Wanna get a quote? Get in touch with us NOW!